One of the language features announced back in PHP 5.6 was the addition of the ... token to denote that a function or method accepts a variable length of arguments.
Something I rarely see mentioned is that it’s possible to combine this feature with type hints to essentially create typed arrays.
For example, we could have a Movie class with a method to set an array of air dates that only accepts DateTimeImmutable objects:
<?php
class Movie {
private $dates = [];
public function setAirDates(\DateTimeImmutable ...$dates) {
$this->dates = $dates;
}
public function getAirDates() {
return $this->dates;
}
}
We can now pass a variable number of separate DateTimeImmutable objects to the setAirDates() method:
<?php
$movie = new Movie();
$movie->setAirDates(
\DateTimeImmutable::createFromFormat('Y-m-d', '2017-01-28'),
\DateTimeImmutable::createFromFormat('Y-m-d', '2017-02-22')
);
If we were to pass something else than a DateTimeImmutable, a string for example, a fatal error would be thrown:
If we instead already had an array of DateTimeImmutable objects that we wanted to pass to setAirDates(), we could again use the ... token, but this time to unpack them:
<?php
$dates = [
\DateTimeImmutable::createFromFormat('Y-m-d', '2017-01-28'),
\DateTimeImmutable::createFromFormat('Y-m-d', '2017-02-22'),
];
$movie = new Movie();
$movie->setAirDates(...$dates);
If the array were to contain a value that is not of the expected type, we would still get the fatal error mentioned earlier.
Additionally, we can use scalar types the same way starting from PHP 7. For example, we can add a method to set a list of ratings as floats on our Movie class:
<?php
declare(strict_types=1);
class Movie {
private $dates = [];
private $ratings = [];
public function setAirDates(\DateTimeImmutable ...$dates) { /* ... */ }
public function getAirDates() : array { /* ... */ }
public function setRatings(float ...$ratings) {
$this->ratings = $ratings;
}
public function getAverageRating() : float {
if (empty($this->ratings)) {
return 0;
}
$total = 0;
foreach ($this->ratings as $rating) {
$total += $rating;
}
return $total / count($this->ratings);
}
}
Again, this ensures that the ratings property will always contain floats without us having to loop over all the contents to validate them. So now we can easily do some math operations on them in getAverageRating(), without having to worry about invalid types.
Problems with This Kind of Typed Arrays
One of the downsides of using this feature as typed arrays is that we can only define one such array per method. Let’s say we wanted to have a Movie class that expects a list of air dates together with a list of ratings in the constructor, instead of setting them later via optional methods. This would be impossible with the method used above.
Another problem is that when using PHP 7, the return types of our get() methods would still have to be “array”, which is often too generic.
Solution: Collection Classes
To fix both problems, we can simply inject our typed arrays inside so-called “collection” classes. This also improves our separation of concerns, because we can now move the calculation method for the average rating to the relevant collection class:
<?php
declare(strict_types=1);
class Ratings {
private $ratings;
public function __construct(float ...$ratings) {
$this->ratings = $ratings;
}
public function getAverage() : float {
if (empty($this->ratings)) {
return 0;
}
$total = 0;
foreach ($this->ratings as $rating) {
$total += $rating;
}
return $total / count($this->ratings);
}
}
Notice how we’re still using a list of typed arguments with a variable length in our constructor, which saves us the trouble of looping over each rating to check its type.
Continue reading %Creating Strictly Typed Arrays and Collections in PHP%
Читать дальше...
Something I rarely see mentioned is that it’s possible to combine this feature with type hints to essentially create typed arrays.
For example, we could have a Movie class with a method to set an array of air dates that only accepts DateTimeImmutable objects:
<?php
class Movie {
private $dates = [];
public function setAirDates(\DateTimeImmutable ...$dates) {
$this->dates = $dates;
}
public function getAirDates() {
return $this->dates;
}
}
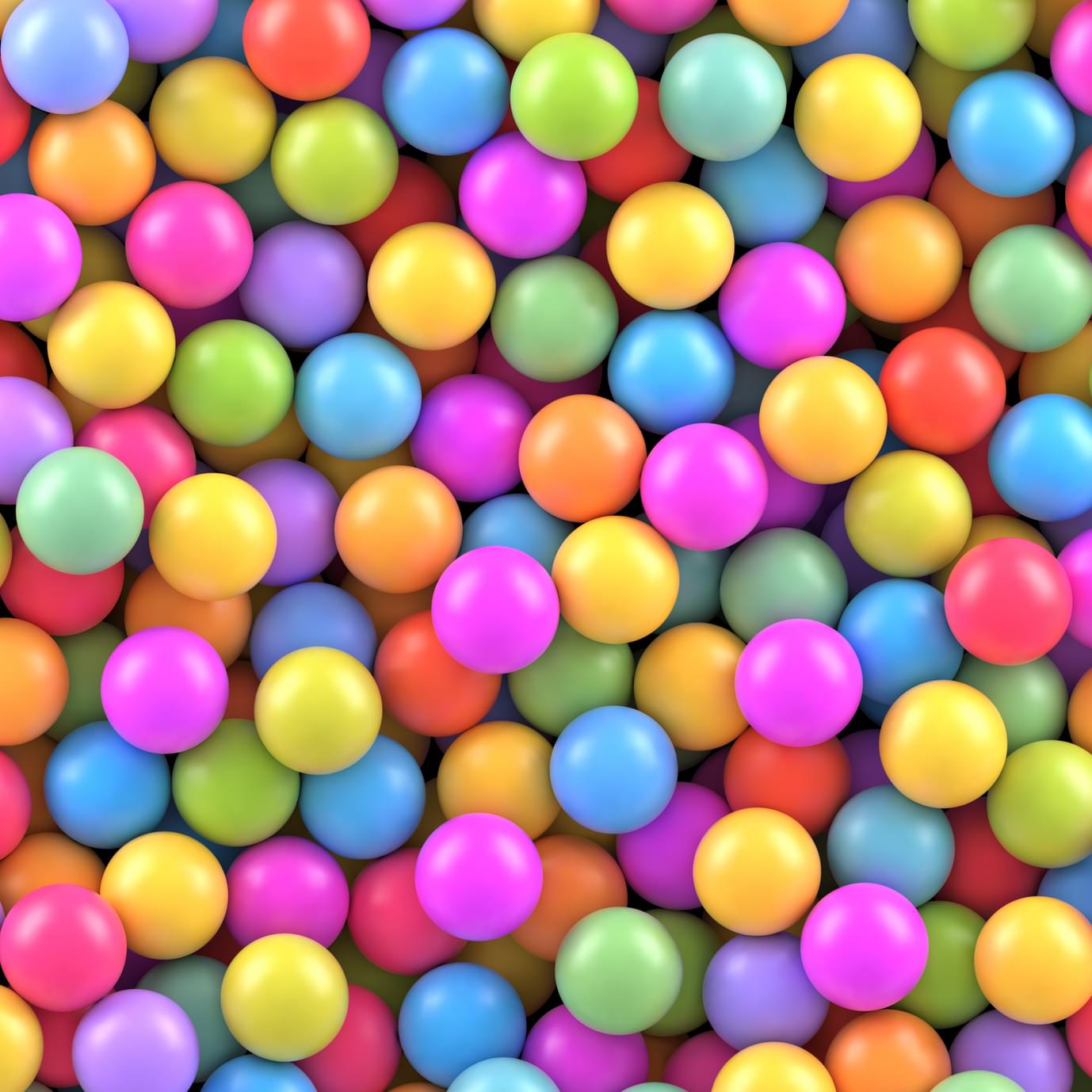
We can now pass a variable number of separate DateTimeImmutable objects to the setAirDates() method:
<?php
$movie = new Movie();
$movie->setAirDates(
\DateTimeImmutable::createFromFormat('Y-m-d', '2017-01-28'),
\DateTimeImmutable::createFromFormat('Y-m-d', '2017-02-22')
);
If we were to pass something else than a DateTimeImmutable, a string for example, a fatal error would be thrown:
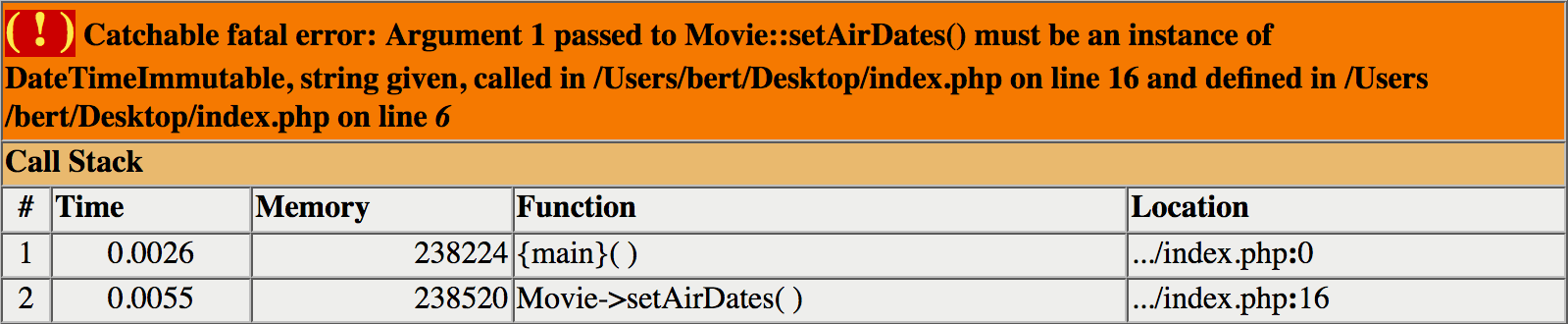
If we instead already had an array of DateTimeImmutable objects that we wanted to pass to setAirDates(), we could again use the ... token, but this time to unpack them:
<?php
$dates = [
\DateTimeImmutable::createFromFormat('Y-m-d', '2017-01-28'),
\DateTimeImmutable::createFromFormat('Y-m-d', '2017-02-22'),
];
$movie = new Movie();
$movie->setAirDates(...$dates);
If the array were to contain a value that is not of the expected type, we would still get the fatal error mentioned earlier.
Additionally, we can use scalar types the same way starting from PHP 7. For example, we can add a method to set a list of ratings as floats on our Movie class:
<?php
declare(strict_types=1);
class Movie {
private $dates = [];
private $ratings = [];
public function setAirDates(\DateTimeImmutable ...$dates) { /* ... */ }
public function getAirDates() : array { /* ... */ }
public function setRatings(float ...$ratings) {
$this->ratings = $ratings;
}
public function getAverageRating() : float {
if (empty($this->ratings)) {
return 0;
}
$total = 0;
foreach ($this->ratings as $rating) {
$total += $rating;
}
return $total / count($this->ratings);
}
}
Again, this ensures that the ratings property will always contain floats without us having to loop over all the contents to validate them. So now we can easily do some math operations on them in getAverageRating(), without having to worry about invalid types.
Problems with This Kind of Typed Arrays
One of the downsides of using this feature as typed arrays is that we can only define one such array per method. Let’s say we wanted to have a Movie class that expects a list of air dates together with a list of ratings in the constructor, instead of setting them later via optional methods. This would be impossible with the method used above.
Another problem is that when using PHP 7, the return types of our get() methods would still have to be “array”, which is often too generic.
Solution: Collection Classes
To fix both problems, we can simply inject our typed arrays inside so-called “collection” classes. This also improves our separation of concerns, because we can now move the calculation method for the average rating to the relevant collection class:
<?php
declare(strict_types=1);
class Ratings {
private $ratings;
public function __construct(float ...$ratings) {
$this->ratings = $ratings;
}
public function getAverage() : float {
if (empty($this->ratings)) {
return 0;
}
$total = 0;
foreach ($this->ratings as $rating) {
$total += $rating;
}
return $total / count($this->ratings);
}
}
Notice how we’re still using a list of typed arguments with a variable length in our constructor, which saves us the trouble of looping over each rating to check its type.
Continue reading %Creating Strictly Typed Arrays and Collections in PHP%
Читать дальше...