Planet PHP
Guest
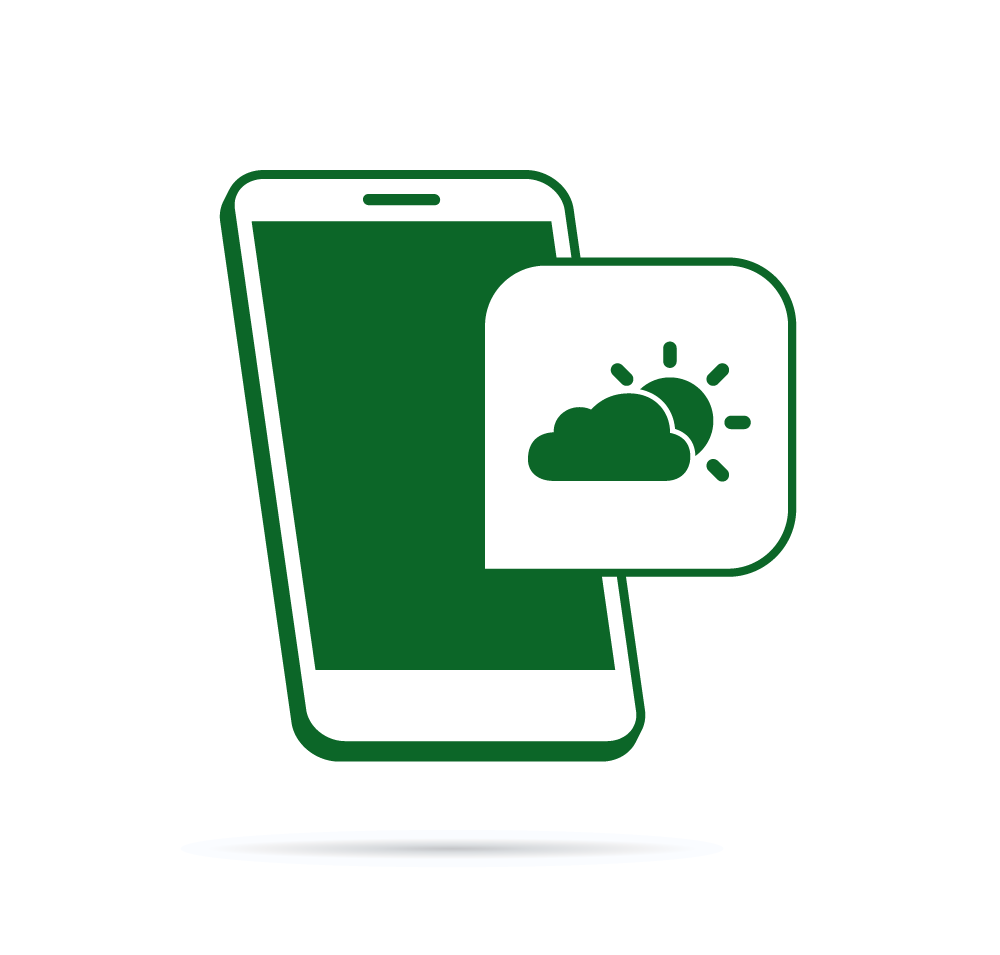
Twilio is a SaaS application which enables developers to build telephone applications using web technologies. In this two-part series, we will leverage Twilio to build a weather forecast app that is accessed using the telephone system. The backend will be written with the Laravel framework (an exploratory video course is available for purchase here, or in the form of written tutorials here).
In this part, we will create a simple program that will allow a user to call a phone number that we buy from Twilio, enter a zipcode, and receive the current weather forecast. The user can also get the weather for any day of the week via the voice menu prompts. In the second part of this series, we will leverage what was built in this article to allow the user to interact with the app via SMS (text message).
Prerequisites
Development Environment
This article assumes Homestead Improved is installed. It is not necessary to use it, but the commands might differ slightly if you use a different environment. If you are not familiar with Homestead and want to produce similar results as this article aims to produce, please visit this SitePoint article that shows how to set up Homestead, and if you need a crash course in Vagrant, please see this post. Additionally, if this whets your appetite and you feel like exploring PHP development environments in depth, we have a book about that available for purchase.
Dependencies
We will create a new Laravel project and then add the Twilio PHP SDK and Guzzle HTTP client library to the project:
cd ~/Code
composer create-project --prefer-dist laravel/laravel Laravel 5.4.*
cd Laravel
composer require "twilio/sdk:^5.7"
composer require "guzzlehttp/guzzle:~6.0"
Development
Let's go through all the steps, one by one.
Routes
Open up the routes/web.php file and add the following ones:
Route::group(['prefix' => 'voice', 'middleware' => 'twilio'], function () {
Route:

Route:

Route:

Route:

});
In this app, all requests will be under the /voice path. When Twilio first connects to the app, it will go to /voice/enterZipcode via HTTP POST. Depending on what happens in the telephone call, Twilio will make requests to other endpoints. This includes /voice/zipcodeWeather for providing today's forecast, /voice/dayWeather, for providing a particular day's forecast, and /voice/credits for providing information on where the data came from.
Service Layer
We are going to add a service class. This class will hold a lot of the business logic that will be shared between the voice telephone app and the SMS app.
Create a new sub-folder called Services inside the app folder. Then, create a file called WeatherService.php and put the following content into it:
<?php
namespace App\Services;
use Illuminate\Support\Facades\Cache;
use Twilio\Twiml;
class WeatherService
{
}
This is a large file in the project, so we will build it piece by piece. Put the following pieces of code in this section inside our new service class:
public $daysOfWeek = [
'Today',
'Sunday',
'Monday',
'Tuesday',
'Wednesday',
'Thursday',
'Friday',
'Saturday'
];
We will use this array to map a day of the week to a number; Sunday = 1, Monday = 2, etc.
public function getWeather($zip, $dayName)
{
Truncated by Planet PHP, read more at the original (another 6325 bytes)
Читать дальше...