Planet PHP
Guest
In the previous post, we saw how to add the follow functionality to a Laravel app. We also looked at how to configure our app to use Stream. This part will focus on:
Activity Fields
When using Stream, models are stored in feeds as activities. An activity is composed of at least the following data fields: actor, verb, object, time. You can also add more custom data if needed.
Let's define the activity verb inside our Post model:
[...]
class Post extends Model
{
[...]
/**
* Stream: Change activity verb to 'created':
*/
public function activityVerb()
{
return 'created';
}
}
Feed Manager
We'll leverage the FeedManager to make our app lively. Stream Laravel comes with a FeedManager class that helps with all common feed operations. We can get an instance of the manager with FeedManager which we set as the facade alias earlier inside the config/app.php file.
Pre-Bundled Feeds
To get us started, the manager has feeds pre-configured. We could also add more feeds if our app needed them. The three feeds are divided into three categories: User Feed, News Feed and Notification Feed. The User feed, for example, stores all activities for a user. Let's think of it as our personal Facebook page. We can easily get this feed from the manager.
For this application, however, we are more interested in getting notifications for posts created by people we follow and also notifications for new follows, thus we'll just stick to the News Feed and the Notification Feed. For more information on the other types of feeds and how to use them visit this link.
Follow / Unfollow Functionality – Using FeedManager
We need to update the follow and unfollow methods inside the FollowController, to take note of the FeedManager:
app/Http/Controllers/FollowController.php
[...]
public function follow(User $user)
{
if (!Auth::user()->isFollowing($user->id)) {
// Create a new follow instance for the authenticated user
Auth::user()->follows()->create([
'target_id' => $user->id,
]);
\FeedManager::followUser(Auth::id(), $user->id);
return back()->with('success', 'You are now friends with '. $user->name);
} else {
return back()->with('error', 'You are already following this person');
}
}
public function unfollow(User $user)
{
if (Auth::user()->isFollowing($user->id)) {
$follow = Auth::user()->follows()->where('target_id', $user->id)->first();
\FeedManager::unfollowUser(Auth::id(), $follow->target_id);
$follow->delete();
return back()->with('success', 'You are no longer friends with '. $user->name);
} else {
return back()->with('error', 'You are not following this person');
}
}
[...]
This code inside the follow method lets the current user's timeline and timeline_aggregated feeds follow another user's personal feed. Inside the unfollow method, we unsubscribe from another user's personal feed.
Continue reading %Building a Social Network with Laravel and Stream? Easy!%
Читать дальше...
- configuring our models in order to make it possible to track activities.
- the different types of feeds that Stream provides.
- getting feeds from Stream.
- rendering the different types of feeds in a view.
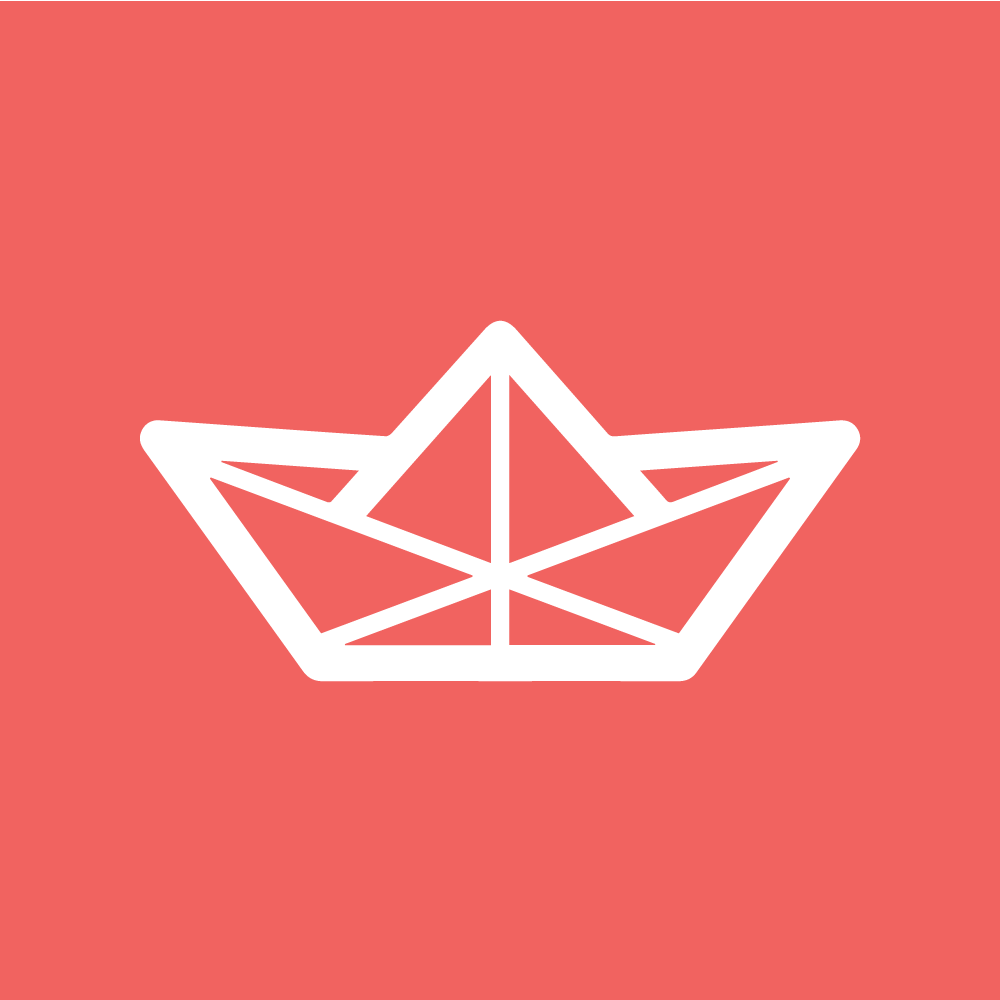
Activity Fields
When using Stream, models are stored in feeds as activities. An activity is composed of at least the following data fields: actor, verb, object, time. You can also add more custom data if needed.
- object is a reference to the model instance itself
- actor is a reference to the user attribute of the instance
- verb is a string representation of the class name
Let's define the activity verb inside our Post model:
[...]
class Post extends Model
{
[...]
/**
* Stream: Change activity verb to 'created':
*/
public function activityVerb()
{
return 'created';
}
}
Feed Manager
We'll leverage the FeedManager to make our app lively. Stream Laravel comes with a FeedManager class that helps with all common feed operations. We can get an instance of the manager with FeedManager which we set as the facade alias earlier inside the config/app.php file.
Pre-Bundled Feeds
To get us started, the manager has feeds pre-configured. We could also add more feeds if our app needed them. The three feeds are divided into three categories: User Feed, News Feed and Notification Feed. The User feed, for example, stores all activities for a user. Let's think of it as our personal Facebook page. We can easily get this feed from the manager.
For this application, however, we are more interested in getting notifications for posts created by people we follow and also notifications for new follows, thus we'll just stick to the News Feed and the Notification Feed. For more information on the other types of feeds and how to use them visit this link.
Follow / Unfollow Functionality – Using FeedManager
We need to update the follow and unfollow methods inside the FollowController, to take note of the FeedManager:
app/Http/Controllers/FollowController.php
[...]
public function follow(User $user)
{
if (!Auth::user()->isFollowing($user->id)) {
// Create a new follow instance for the authenticated user
Auth::user()->follows()->create([
'target_id' => $user->id,
]);
\FeedManager::followUser(Auth::id(), $user->id);
return back()->with('success', 'You are now friends with '. $user->name);
} else {
return back()->with('error', 'You are already following this person');
}
}
public function unfollow(User $user)
{
if (Auth::user()->isFollowing($user->id)) {
$follow = Auth::user()->follows()->where('target_id', $user->id)->first();
\FeedManager::unfollowUser(Auth::id(), $follow->target_id);
$follow->delete();
return back()->with('success', 'You are no longer friends with '. $user->name);
} else {
return back()->with('error', 'You are not following this person');
}
}
[...]
This code inside the follow method lets the current user's timeline and timeline_aggregated feeds follow another user's personal feed. Inside the unfollow method, we unsubscribe from another user's personal feed.
Continue reading %Building a Social Network with Laravel and Stream? Easy!%
Читать дальше...